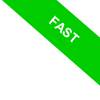
The __delattr__() Method in Python
The special method `__delattr__()` in Python allows you to delete an attribute from an object.
It’s a concept that might not be immediately clear to beginners, but once understood, it can be quite useful.
In Python, everything is an object, and every object has attributes (properties, methods, etc.).
For example, consider this class definition:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Here, `name` and `age` are attributes of the object created from the `Person` class.
Let’s create an instance of the class:
p = Person("Anna", 30)
You can easily access and modify these attributes:
print(p.name)
Anna
You can also remove an attribute from the object using the `del` statement:
del p.name
When you execute `del p.name`, Python internally calls the method `__delattr__(self, name)`.
It’s somewhat similar to how you would use `__getattr__()` to access an attribute or `__setattr__()` to set one.
In fact, you can achieve the same result by calling the `__delattr__()` method directly on the object:
p.__delattr__('name')
In both cases, the 'name' attribute is deleted from the object 'p'.
print(p.name)
AttributeError: 'Person' object has no attribute 'name'
The true power of the `__delattr__()` method, however, lies in its ability to customize attribute deletion within a class.
Let’s rewrite the class, this time customizing the `__delattr__()` method:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __delattr__(self, attr):
print(f"About to delete the attribute {attr}")
super().__delattr__(attr)
Now, create an instance of the class:
p = Person("Anna", 30)
Then, delete the 'name' attribute:
del p.name
In this case, Python calls the `__delattr__()` method you’ve overridden in the 'Person' class, printing a message before the attribute is actually removed.
Notice that we use `super().__delattr__(attr)` to ensure the default behavior is preserved when deleting the attribute. Without this, the method would recursively call itself indefinitely.
Why customize attribute deletion?
Imagine you’re working in a system where deleting an attribute could have significant consequences or requires specific checks.
For instance, you might want to ensure it’s safe to remove an attribute, or you might need to log the operation.
Here’s an example of how you could extend the 'Person' class:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __delattr__(self, attr):
if attr == "name":
# Prevent the deletion of the 'name' attribute
print("You cannot delete the 'name' attribute!")
else:
super().__delattr__(attr) # Allow deletion of other attributes
In this case, the `__delattr__()` method prevents the deletion of the 'name' attribute.
Create an object from the 'Person' class:
p = Person("Anna", 30)
Now, try to delete the 'name' attribute:
del p.name
Python will now stop you from deleting the attribute:
You cannot delete the 'name' attribute!
In summary, while you might not need the `__delattr__()` method frequently, it’s important to know that it exists. This method provides you with control over how attributes are deleted, allowing for more sophisticated behavior when necessary.