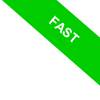
Class Properties in Python
Today, we're diving into class properties in Python. Properties are attributes that hold values, information, or data within classes.
What's the difference between properties and methods? While both are class attributes, properties and methods serve different purposes. Properties manage access to a class's data attributes. For instance, a property in the "Car" class could specify the car's "color." On the other hand, methods are functions tied to a class that define its behaviors and operations. In the "Car" class, a method could be an action like "turn_right()".
In Python, properties act as non-callable attributes.
They are useful for storing data within objects created from a class.
There are designated methods to retrieve a property's value (getter) or to alter it (setter).
Python also includes callable properties, which are essentially methods that function as properties. We will explore this further in a dedicated section on classes.
Let's explore this with a practical example.
Consider a `Person` class with two properties: `name` and `age`.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Once defined, you can create instances of the class.
For example, to instantiate a 'Student' object with the name "Mary" and age 30:
Student = Person("Mary",30)
Accessing properties is straightforward—simply append the property name to the object name, separated by a dot.
For example, you can access the `age` property like so:
print(Studente.age)
30
To denote a private attribute, it's common practice to prefix its name with an underscore, signaling that it should not be accessed directly.
Let’s modify the earlier class to make the `age` property private:
class Person:
def __init__(self, name, age):
self.name = name
self._age = age
@property
def age(self):
"""Getter for age."""
return self._age
Student = Person("Mary", 30)
print(Student.age)
In this example, `_age` is a "private" attribute used to store the age value discreetly.
The `age` property, defined with the `@property` decorator, facilitates the retrieval of `_age` through a getter method, maintaining the output consistently.
30
The use of @property offers numerous advantages, such as encapsulation. This feature shields the implementation details while exposing a safe interface, allowing for modifications in the backend without altering how the class is used externally.
To ensure that the age is never negative, we can introduce a setter method that oversees modifications to the `_age` property.
class Person:
def __init__(self, name, age):
self.name = name
self._age = age
@property
def age(self):
"""Getter for age that prevents negative values."""
return self._age
@age.setter
def age(self, value):
"""Setter for age that ensures values are non-negative."""
if value < 0:
raise ValueError("Age cannot be negative.")
self._age = value
Student = Person("Mary", 30)
Student.age=-30
print(Student.age)
This approach allows us to treat `age` as a normal attribute, but with built-in safeguards.
Attempting to set a negative age triggers an exception, reinforcing data integrity.
Age cannot be negative.
As demonstrated, a setter method for the `age` property ensures data validity before it's officially set, proving essential for maintaining the robustness of your data model.
The setter method also proves invaluable when dealing with attributes that necessitate complex calculations. It can be paired with a property that calculates only as needed. For instance, changing the `age` property could trigger an update to another attribute that calculates the years remaining until retirement.
class Person:
def __init__(self, name, age):
self.name = name
self._age = age
@property
def age(self):
"""Getter for age."""
return self._age
@age.setter
def age(self, value):
"""Setter for age that prevents negative values."""
if value < 0:
raise ValueError("Age cannot be negative.")
& nbsp; self._age = value
# Update a related attribute when the age changes
self._years_to_retirement = 65 - self._age if self._age < 65 else 0
Student = Person("Mary", 30)
print(Student.age)
Properties in Python are indeed a potent tool that can greatly enhance the safety and maintainability of your code.
Mastery of these features can significantly uplift your class designs.