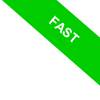
The __bases__ Attribute in Python
The `__bases__` attribute of classes lets us delve into class inheritance, a fundamental concept in object-oriented programming (OOP).
class.__bases__
The `__bases__` attribute of a class is a tuple that contains the base classes (or superclasses) from which the current class inherits.
In other words, it tells us which classes our class has inherited from.
In Python, everything is an object, including classes, because every class in Python is an instance of the metaclass `type`. Understanding which classes a class inherits from can be very useful, especially when working with complex code or third-party libraries. For instance, we can use `__bases__` to build a function that recursively explores the class hierarchy:
Let's take a closer look at what this means and how we can use it in practice.
Imagine you have a simple class hierarchy:
class A:
pass
class B(A):
pass
class C(B,A):
pass
In this hierarchy, class `B` inherits from `A`, and class `C` inherits from both `B` and `A`.
Let's see what the `__bases__` attribute reveals for each of these classes:
Class `A` inherits directly from `object`, which is the base class for all classes in Python.
print(A.__bases__)
(<class 'object'>,)
Class `B` inherits from `A`.
print(B.__bases__)
(<class '__main__.A'>,)
Class `C` inherits from both `B` and `A`.
print(C.__bases__)
(<class '__main__.B'>, <class '__main__.A'>)
What is the Difference Between the __base__ and __bases__ Attributes?
In brief, the difference between these two similar attributes is as follows:
- __base__ returns the closest direct base class of a class. If the class inherits from multiple classes, __base__ returns only the first direct base class according to the Method Resolution Order (MRO).
- __bases__ returns a tuple containing all the direct base classes from which a class inherits. If the class inherits from multiple classes, __bases__ includes them all.
How to List Base Classes in Hierarchical Order
To see the list of base classes a class inherits from, in hierarchical order, you can define this function:
def print_class_hierarchy(cls, level=0):
indent = ' ' * (level * 4)
print(f"{indent}{cls.__name__}")
for base in cls.__bases__:
print_class_hierarchy(base, level + 1)
In this example, the `print_class_hierarchy` function prints the class hierarchy starting from a given class.
For example, call this function on class `C`.
print_class_hierarchy(C)
The function returns the class hierarchy that class `C` depends on.
The indentation helps us clearly visualize the levels of inheritance.
C
B
A
object
In conclusion, the `__bases__` attribute allows you to understand from which classes a class inherits.
This is especially useful in complex contexts such as large libraries or exception hierarchies.