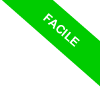
Class Inheritance in Python
Class inheritance is a core concept in object-oriented programming (OOP) that allows a class, called a derived class or subclass, to inherit attributes and methods from another class, called a base class or superclass.
Why is it useful? Inheritance lets you reuse code you have already written, which reduces the need for rewriting and makes it easier to maintain and expand functionality. It also helps organize your code structure in a more modular way.
To better understand how inheritance works, let's consider a practical example in Python.
Imagine you have a class `Animal` that represents a generic animal with a name and a method to make a sound:
class Animal:
def __init__(self, name: str):
self.name = name
def sound(self):
return "Some generic sound"
Now, create a class `Dog` that represents a dog.
Instead of redefining everything from scratch, you can make `Dog` inherit from `Animal` and add its own specific `sound` method:
class Dog(Animal):
def sound(self):
return "Woof"
In this example, the `Dog` class inherits the `name` attribute from the `Animal` class. Additionally, it overrides the `sound` method to return a dog-specific sound.
This way, you avoid having to redefine them again.
Now, create an instance of the `Dog` class:
dog = Dog("Buddy")
If you query the object's "name" attribute, you get the name you assigned to it:
print(dog.name)
Buddy
If you call the `sound()` method, you get the dog's sound:
print(dog.sound())
Woof
To make the example even clearer, add a second derived class, `Cat`, representing a cat:
class Cat(Animal):
def sound(self):
return "Meow"
In this case, you have created a `Cat` class that inherits from `Animal` and overrides the `sound` method to return a cat-specific sound.
For example, create an instance of the `Cat` class:
cat = Cat("Whiskers")
Then call the object's `sound()` method:
print(cat.sound())
Meow
This simple example shows how inheritance allows you to reuse and extend code effectively.
You can also use classes to define attributes of other classes
Here's an example where an attribute of one class is defined using another external class.
This example demonstrates object composition, another important concept in object-oriented programming.
Imagine you have a class `Address` that represents an address:
class Address:
def __init__(self, street: str, city: str, zipcode: str):
self.street = street
self.city = city
self.zipcode = zipcode
def __str__(self):
return f"{self.street}, {self.city}, {self.zipcode}"
Then create a `Person` class that uses `Address` as one of its attributes:
class Person:
def __init__(self, name: str, surname: str, address: Address):
self.name = name
self.surname = surname
self.address = address
def __str__(self):
return f"{self.name} {self.surname}, Address: {self.address}"
Now create an instance of `Address` and use it to create an instance of `Person`:
home_address = Address("123 Main St", "Springfield", "12345")
Next, create an instance of the `Person` class using the `Address` instance:
person = Person("John", "Doe", home_address)
If you now query the object's attributes, you will see that it has inherited the address structure:
print(person)
John Doe, Address: 123 Main St, Springfield, 12345
In this example, the `Person` class has an `address` attribute that is an instance of the `Address` class.
This demonstrates how one class can use another class as part of its definition, promoting modularity and code reuse.