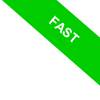
The __del__() Method in Python
Let's explore the __del__() method in Python. This special method, also known as the destructor method, is invoked when an object is about to be destroyed by Python's garbage collector—essentially when no more references to that object exist.
In Python, the __del__() method is part of the special methods (often referred to as magic methods), similar to __new__() and __init__(). While the constructor __init__() is called when an object is created, the __del__() method is called when an object is deleted using the del statement or when there are no remaining references to it.
This method is particularly useful for executing any necessary cleanup code before the object is destroyed.
It allows you to create a custom destructor, which is helpful for tasks like closing open files, freeing up network resources, or disconnecting from a database.
It's important to note that the __del__() method is not defined in the object class, the base class of all classes, so it is not available by default in any class.
When should you use it? The __del__() method is not commonly used because Python handles most cleanup operations automatically through its garbage collector. However, there are scenarios where you may want to ensure specific actions are taken when an object is no longer in use.
Let’s consider a practical example.
Suppose we have a class that represents a file connection.
We want to ensure that when the object is destroyed, the file is properly closed. Here’s how you might implement it:
class FileHandler:
def __init__(self, filename):
self.filename = filename
self.file = open(filename, 'w')
print(f"File '{self.filename}' opened.")
def write_data(self, data):
self.file.write(data)
print(f"Data written to '{self.filename}'.")
def __del__(self):
self.file.close()
print(f"File '{self.filename}' closed.")
Now, let’s create an instance of the FileHandler class:
handler = FileHandler('example.txt')
handler.write_data("Hello, world!")
When the handler object is no longer needed, the file will be closed automatically.
You can also manually close the file using the del statement, which triggers the __del__() method:
del handler
In this example, when the handler object is destroyed by executing del handler, the __del__() method is called, ensuring that the open file is closed properly.
This process helps prevent issues like locked files or data loss by ensuring all resources are released.
Here’s another example of how the __del__() method works:
Define this class:
class Foo:
def __del__(self):
print(self, 'is about to be destroyed.')
Create an instance of the class:
a = Foo()
If you assign a new value to the variable a, the garbage collector will delete the object because it no longer has any references.
a = 10
In this case, the __del__() method is automatically executed.
<__main__.Foo object at 0x7237d55e3f40> is about to be destroyed.
However, as we’ll see, the execution of the __del__() method doesn’t necessarily mean the object will be immediately deleted by the garbage collector.
Challenges with the __del__() Method
Before using this method, it's important to consider some critical aspects:
- The garbage collector might not call the destructor
Unlike other programming languages, Python doesn’t guarantee when the __del__() method will be called. This depends on the garbage collector’s implementation, and it might not happen immediately after an object is no longer in use. - Cyclic references
Having a __del__() method can complicate an object’s lifecycle, especially if there are cyclic references. Python may have difficulty correctly destroying objects if reference cycles involve objects with a __del__() method. For example:
class A:
Create instances of the classes:
def __init__(self):
self.b = None
print("A: object created")
def __del__(self):
print("A: object destroyed")
class B:
def __init__(self):
self.a = None
print("B: object created")
def __del__(self):
print("B: object destroyed")a = A()
Now create a mutual reference:
b = B()a.b = b
In this situation, the __del__() method might not be called when you run del a or del b.
b.a = aUpdate: The issue with cyclic references was resolved starting with Python 3.4. From this version onward, the __del__() method is called consistently even if two objects reference each other.
In conclusion, while the __del__() method can be useful for ensuring resource cleanup, it should be used cautiously.
Python provides more reliable tools, such as context managers, to handle most cleanup tasks.
If you need to release resources in a more predictable manner, consider using context managers like the with statement along with the __enter__() and __exit__() methods instead of __del__(). These approaches are more dependable for managing resource cleanup.
So, while it’s important to understand __del__(), exploring more robust alternatives