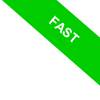
Methods in a Python Class
Methods are functions within a class that define how objects of that class behave or how the class itself operates.
What is a class? In Python, think of a class as a blueprint for creating objects that share a set of properties and behaviors.
Class attributes in Python are typically divided into two categories: methods and properties.
- Methods (callable attributes)
Defined as functions within the class, these attributes are "callable," meaning you can invoke them like any other function. They process and manipulate the data of an object or modify the state of the class itself. - Properties (non-callable attributes)
These attributes are static and hold data. They are usually initialized in the class's __init__ method and help maintain the object's state. Common properties might include attributes like name, brand, or model in a class that represents a product or vehicle.
Generally, methods can be thought of as the callable attributes of a class.
Python also recognizes a third category known as "callable properties," which allows methods to function as non-callable attributes. This topic will be explored in more detail in a future lesson.
The Python language supports various types of methods including instance methods, class methods, and static methods.
Instance and class methods are tied to either the instance or the class itself, and are known as "bound methods."
In contrast, static methods are independent of both the instance and the class.
Instance Methods
Instance methods are used to manipulate the data of an individual object, an instance of the class.
These methods require a reference to the specific object (`self`) to interact with its data.
class Automobile:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def describe(self):
return f"{self.brand} {self.model}"
# Creating an instance
car = Automobile("Fiat", "500")
print(car.describe())
Fiat 500
This `describe` method is a classic example of an instance method, utilizing `self` to provide a descriptive summary of the car.
Class Methods
Class methods affect the class as a whole rather than individual instances.
These are identified by the `@classmethod` decorator and accept the class itself as the first argument, typically named `cls`.
class Automobile:
tax_rate = 1.20
@classmethod
def calculate_tax(cls, price):
return cls.tax_rate * price
# Using the class method
print(Automobile.calculate_tax(15000))
18000
The `calculate_tax` method demonstrates how a class method can be used to apply a class-wide tax rate to a given price.
Static Methods
Static methods, marked with the `@staticmethod` decorator, do not automatically access the instance (`self`) or the class (`cls`). They are useful for tasks that are independent of the class's state.
class Automobile:
@staticmethod
def validate_plate(plate):
return len(plate) == 7
# Using the static method
print(Automobile.validate_plate("1234567"))
True
This method provides a practical example of a static method's utility in verifying whether a license plate contains the correct number of characters.
As you design a class, think strategically about which functions are essential to instances, which should be managed at the class level, and which are general utilities that stand apart from specific instances or the class structure. This approach will help you organize your code more effectively, ensuring it is both manageable and comprehensible.
Local Variables in Methods
Variables declared within methods are local variables specific to those methods.
These variables are not accessible outside the method or by other instances/methods unless they are defined as instance or class attributes.
This setup ensures that variables within methods exist only during the execution of the method and are not visible or accessible outside of it. Such isolation maintains method independence and supports encapsulation. Encapsulation is a cornerstone of object-oriented programming, safeguarding an object’s internal state from external access while exposing only necessary details through public methods.
For example, the following script includes a class with several variables of different types:
class Car:
message="welcome" # Class attribute
def __init__(self, color):
self.color = color # Instance attribute
self.message="hello"
def display_info(self):
message = f"This car is {self.color}." # Local variable
return message
# Instances of the Car class
car1 = Car("red")
message="welcome" # Global variable
In this script, there are four instances of a variable named "message," each with different scopes: as a local variable in a method, an instance attribute, a class attribute, and a global variable.
In Python, the scope of a variable defines where it can be accessed. Each scope type governs the visibility and life span of the variable within that context.
The local variable "message" is visible only within the display_info(self) method that declares it.
print(car1.display_info())
This car is red.
By contrast, when you query car1.message, Python accesses the "hello" instance attribute.
print(car1.message)
hello
Note that when a method attempts to reference variables not declared within its local scope, it first searches through instance variables, followed by class variables, and then global variables.
If no suitable variable is found, Python raises a NameError, indicating that the variable has not been defined.
Newcomers often make the mistake of trying to access a class variable directly within a method without using the class name or 'self' for instance variables. This oversight usually leads to a NameError unless there is a coinciding local or global variable with the same name.