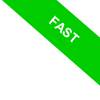
Class Methods in Python
Class methods in Python are a unique type of method that takes the class itself as its first implicit argument, instead of an instance.
These methods are useful when you need to access or modify the class's state rather than that of an individual instance.
To define a class method, use the @classmethod decorator.
class ClassName:
@classmethod
def methodName(cls):
print(id(cls))
The first parameter of the class method is conventionally named cls, representing the class to which the method belongs.
Here is an example to illustrate:
Define a class called "myClass" with a class method named "myMethod".
class myClass:
@classmethod
def myMethod(cls):
print(id(cls))
The myMethod() simply returns the identifier of the class.
Now, call the class method:
myClass.myMethod()
93842345589184
The class identifier is 93842345589184.
To verify, you can use the id() function:
print(id(myClass))
93842345589184
Create an instance of the "myClass" class:
p = myClass()
You can also call the myMethod() class method through the instance:
p.myMethod()
93842345589184
The result remains the same.
Note that even when you call the myMethod class method through an instance of `myClass`, the class is automatically passed as the first argument. This is evident from the printed id, which matches the id of the `myClass` class. Therefore, the cls parameter must always be included when defining a class method.
If you attempt to define a class method without specifying the cls parameter, you'll encounter an error because the class is still passed as the first implicit argument:
For example, define the class method without the cls parameter.
class myClass:
@classmethod
def myMethod():
print('test')
If you now call the myMethod as a class method, Python returns an error.
myClass.myMethod()
TypeError: myClass.myMethod() takes 0 positional arguments but 1 was given
Finally, remember that class methods are recognized as objects of type `method`:
import types
print(type(myClass.myMethod))
<class 'method'>
In summary, class methods provide a way to define behaviors involving the class itself rather than its instances. They are identified by the use of the `@classmethod` decorator and the `cls` parameter.