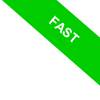
Instance Methods in Python
In Python, instance methods are methods defined within a class that operate on a specific instance of that class.
These methods can access and modify the object's state and interact with other methods and attributes of the same class.
When you call an instance method through an instance of the class, the instance itself is automatically passed as the first argument to the method.
This argument is commonly known as `self`.
class Foo:
def foo(self):
print(self)
Therefore, it's necessary to define instance methods in a class with at least one self parameter that represents the instance on which the method operates, allowing access to its attributes and methods.
Using self, you can access and modify the instance's attributes.
The self parameter must be the first parameter of every instance method, even though it is not explicitly passed when the method is called.
Besides instance methods, there are also class methods and static methods. Class methods use the @classmethod decorator and receive the class itself (cls) as the first parameter, while static methods use @staticmethod and do not receive either the instance or the class as a parameter.
Let's start with a simple example.
Imagine you want to create a class to represent a person. The class will have some attributes, like name and age, and methods to interact with these attributes.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def describe(self):
return f"{self.name} is {self.age} years old."
def age_up(self, years):
self.age += years
The describe(self) method allows you to view the person's "age" attribute.
The age_up(self, years) method allows you to increase the person's age.
The __init__ method is a special method called a "constructor". It is automatically executed when we create a new instance of the class. It initializes the instance's attributes. In this case, name and age are initialized with the values passed as arguments.
Now, create an instance "person1" of the Person class.
person1 = Person("Alice", 30)
Then call the instance method describe()
This method reads and displays the age of the person within the "person1" instance.
print(person1.describe())
Alice is 30 years old.
Finally, call the age_up(5) method.
This method increases the person's age by 5 years within the "person1" instance.
Then call the describe() method again to display the updated information.
person1.age_up(5)
print(person1.describe())
Alice is 35 years old.
As you can see, both methods were called from the "person1" instance and operate within the instance itself.
Why is the self parameter important? When an instance method is called on an object (instance), the object itself is automatically passed as the first argument and stored in the "self" parameter. A common mistake is forgetting to include `self` as the first parameter in the definition of an instance method. In these cases, Python generates an error because it expects the method to accept the instance as the first argument. For example, define the instance method describe() without specifying the self parameter.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def describe():
return f"{name} is {age} years old."
Then create an instance "person1" of the "Person" class and call the describe() method from the instance.
person1 = Person("Alice", 30)
print(person1.describe())
In this case, Python returns an error message.
TypeError: Person.describe() takes 0 positional arguments but 1 was given
The error occurs because the describe() method is not defined to accept the instance (self) as an argument. Therefore, you cannot call it from an instance. So, always remember to include `self` as the first parameter in instance methods to avoid this common error.
At this point, you should know everything you need to work with instance methods in Python.