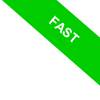
Static Methods in Python
Today we're discussing static methods in Python. While this topic might seem a bit abstract at first, it becomes very useful once you understand it well.
What is a static method? In Python, a static method is a type of method that belongs to a class but cannot directly access instance attributes (like `self`) or class attributes (like `cls`). This means a static method cannot modify the state of the instance or the class. It's like a regular function but is contained within the class's namespace.
In other words, a static method of a class can be called without creating an instance.
In Python, you can define a static method using the @staticmethod decorator.
@staticmethod
def methodName():
pass
Static methods are particularly useful when you want to group utility functions under a class's namespace for better code organization and want them to be callable without instantiating the class.
Additionally, since they are independent of the instance state, static methods can be slightly more efficient in terms of performance.
Class methods and instance methods are objects of types.MethodType, whereas static methods are objects of types.FunctionType, meaning they are straightforward functions. As such, they do not have the __self__ attribute. The __self__ attribute refers to the object implicitly passed as the first argument, this is the instance in instance methods and the class in class methods.
Here's a practical example:
class Calculator:
@staticmethod
def addition(a, b):
return a + b
def subtraction(self, a, b):
return a - b
In this example, the addition() method is a static method of the class.
This means you can call it without creating an instance of the class:
result1 = Calculator.addition(5, 3)
print(result1)
8
The subtraction() method, on the other hand, is an instance method.
Therefore, you can only call it from an instance of the class.
obj = Calculator()
result2 = obj.subtraction(10, 4)
print(result2)
6
This simple example clearly illustrates the difference between static methods and instance methods.
You cannot call the instance method without first defining an object of the class.
Conversely, you can call the static method without defining an instance, and if you want, also from an instance.
For example, define an instance of the class and call the static method addition() from the instance:
obj = Calculator()
result2 = obj.addition(5, 3)
print(result2)
8
Note that you can create a static method implicitly, without using the @staticmethod decorator. However, this has some limitations compared to using the explicit decorator, and the result is not exactly the same.
class Calculator:
def addition(a, b):
return a + b
This allows you to call the method without creating an instance of the class.
result1 = Calculator.addition(5, 3)
print(result1)
8
However, in this case, you cannot call the method from an instance of the class.
obj = Calculator()
result2 = obj.addition(5, 3)
print(result2)
TypeError: Calculator.addition() takes 2 positional arguments but 3 were given
In other words, when you create a static method implicitly, what you define is simply a function housed within the class.
In conclusion, remember that static methods are simply regular functions encapsulated within the class's namespace. Use them only when you need functions that do not depend on the state of an instance or the class itself.
I hope this guide has been helpful. If you have any other questions or want to delve deeper, feel free to ask!