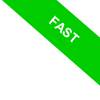
Method Overwriting in Python
In object-oriented programming, method overwriting is also known as overwriting. Let’s explore how this is implemented in Python.
What is overwriting? It’s a technique that allows a derived class (or subclass) to replace a method already defined in its base class (or superclass). The base class method is ignored. As we will see, in Python there is a distinction between overwriting and overriding.
Let’s consider a practical example.
First, define a base class called `Animal` with a method named `sound`:
class Animal:
def sound(self):
print('This animal makes a sound: ')
Now, define a subclass called `Dog` that inherits attributes and methods from the `Animal` class:
class Dog(Animal):
def sound(self):
return "Woof Woof!"
In this implementation, the `sound` method of the `Dog` class overwrites the `sound` method of the `Animal` class.
This is an example of overwriting because the superclass method is completely replaced by the new implementation in the derived class. It is neither called nor extended.
To better understand how overwriting works, create an instance of the `Dog` class:
dog = Dog()
Then, call the sound() method through the instance:
print(dog.sound())
Woof Woof!
As you can see, the `Dog` class provides its own implementation of the `sound` method, replacing that of the base `Animal` class.
Therefore, the initial definition of the method in the `Animal` class is completely ignored.
This allows the subclass to define a new, specific behavior.
Are overwriting and overriding the same thing? Not exactly. They are both useful techniques for customizing methods inherited from a base class, but there are some important differences:
- Overwriting: The subclass provides a new implementation of the method that completely replaces that of the superclass. The superclass method is not called.
- Overriding: The subclass can provide a new implementation of the method and can also call the superclass method using super(), thus extending the original behavior. In other words, in overriding, the method code in the base class is not ignored but extended.
I hope this guide has clarified the concepts of overwriting and method overriding.