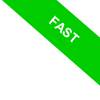
Overriding Class Methods in Python
Overriding is a core concept in object-oriented programming (OOP) in Python. It allows you to extend and redefine the behavior of methods inherited from a base class within a derived class.
Here’s a practical example.
Define a base class called `Animal` that includes a method called sound().
class Animal:
def sound(self):
print("The sound of the animal is:")
Next, define a derived class `Dog` that inherits attributes and methods from the base class `Animal`, but overrides the sound() method by adding a new line of code.
class Dog(Animal):
def sound(self):
super().sound()
return "woof woof"
The super() function allows you to call the base class's sound() method, while adding an extra line of code in the derived class.
For example, create an instance of the `Dog` class:
fido = Dog()
Then call the sound() method on the instance:
print(fido.sound())
The sound of the animal is:
woof woof
The instance executes the sound() method of the base class 'Animal' via the super() function, and then continues with the custom behavior defined in the derived class 'Dog'.
What are the benefits of overriding?
Overriding adapts the behavior of generic methods to specific cases in derived classes, avoiding code duplication since base methods are inherited and then customized as needed.
This enables object polymorphism in Python.
In other words, objects from different classes can respond in unique ways while using the same method from the base class.
The difference between overriding and overwriting. The distinction between these two techniques is subtle due to their similarities. Overriding extends the base class method without replacing it, while overwriting replaces the base class method entirely with one from the derived class.
In conclusion, overriding is particularly useful when you want to specialize inherited behavior without modifying attributes or adding new methods.